In this Arduino tutorial we will learn how to control 8×8 LED Matrix using the MAX7219 driver and the Arduino board. You can watch the following video or read the written tutorial below for more details.
Overview
We will make three examples, with the first one we will explain the basic working principle of the MAX7219 , in the second example we will see how the scrolling text on the 8×8 LED Matrix works, and in the third example we will control them via Bluetooth and a custom build Android application.
MAX7219
Now let’s take a closer look at the MAX7219 driver. The IC is capable of driving 64 individual LEDs while using only 3 wires for communication with the Arduino, and what’s more we can daisy chain multiple drivers and matrixes and still use the same 3 wires.
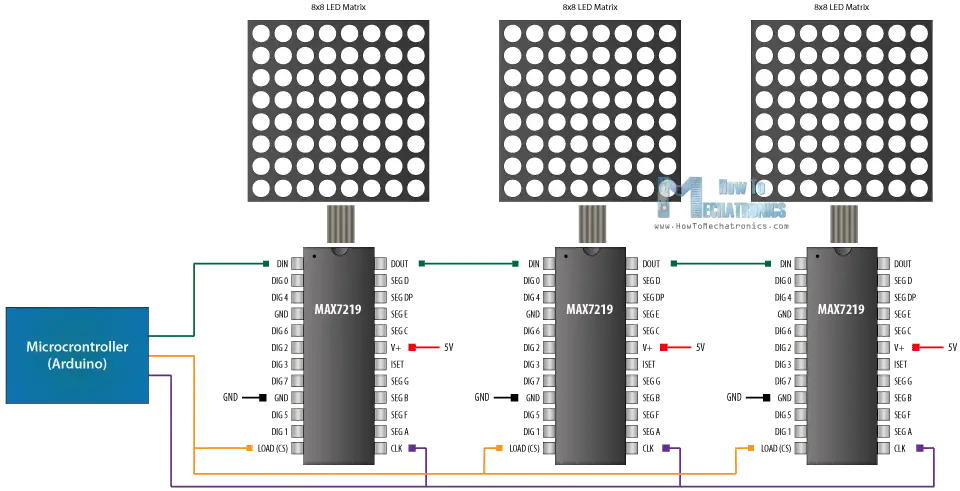
The 64 LEDs are driven by 16 output pins of the IC. The question now is how is that possible. Well the maximum number of LEDs light up at the same time is actually eight. The LEDs are arranged as 8×8 set of rows and columns. So the MAX7219 activates each column for a very short period of time and at the same time it also drives each row. So by rapidly switching through the columns and rows the human eye will only notice a continuous light.
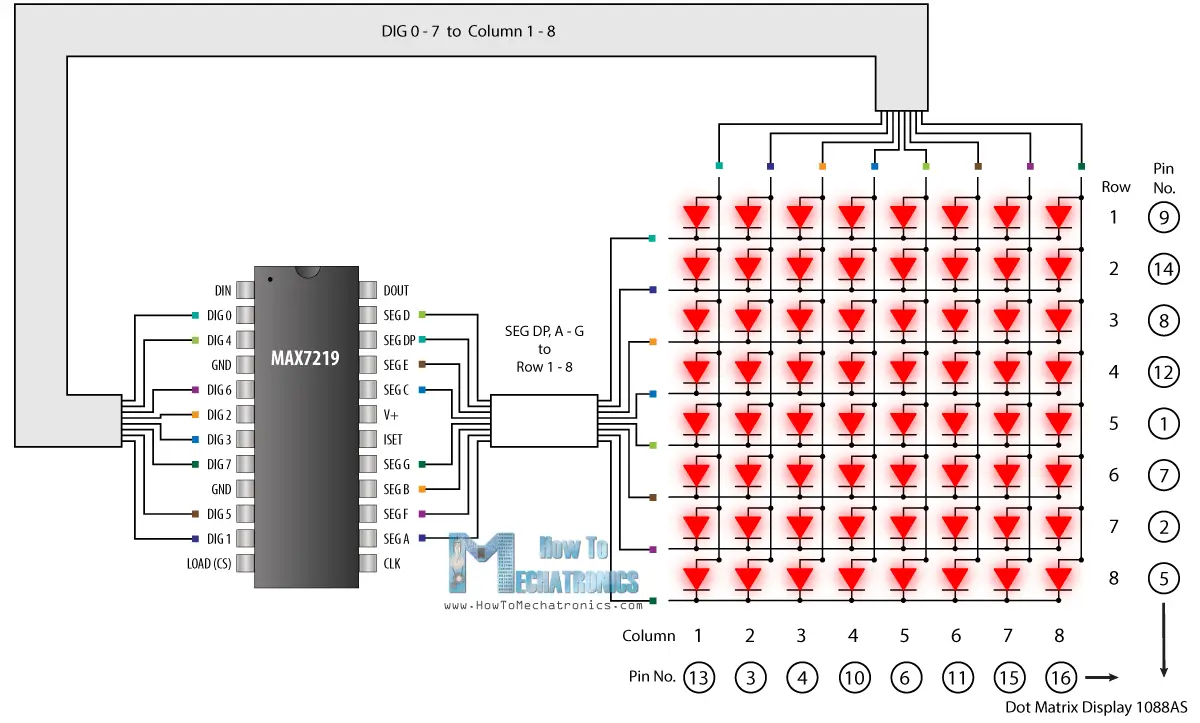
Note how the pins of a common 8×8 LED Matrix are internally arranged, so if you are building a matrix on your own you should consider it. Also note that a common breakout board for the MAX7219 comes with a resistor between the 5V and the IC pin number 18. The resistor is used for setting the brightness or the current flow to the LEDs.
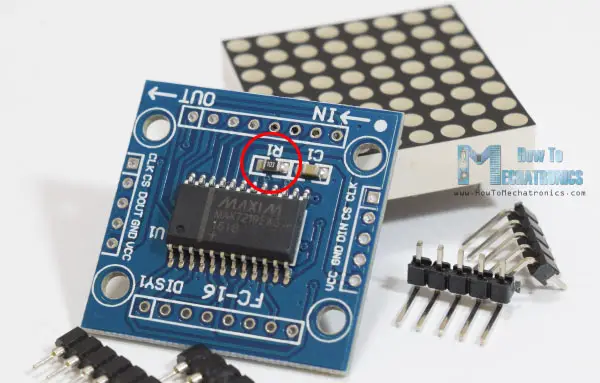
The following table from the datasheet of the IC shows the value of the resistor that we should use according to the forward voltage drop of our LEDs.
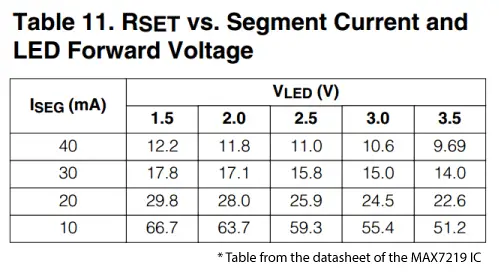
Circuit Schematic
Now let’s connect the 8×8 LED Matrix module to the Arduino Board. Here’s the circuit schematic:
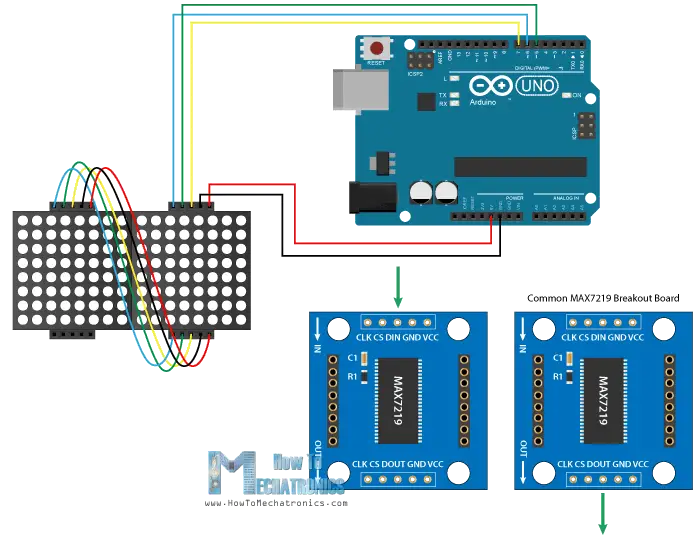
The VCC and GND of the module go to the 5V and GND pins of the Arduino and the three other pins, DIN, CLK and CS go to any digital pin of the Arduino board. If we want to connect more than one module we just connect the output pins of the previous breakout board to the input pins of the new module. Actually these pins are all the same except that the DOUT pin of the previous board goes to the DIN pin of the new board.
You can get the components needed for this Arduino Tutorial from the links below:
- 8×8 LED MAX7219 Dot Matrix Module…… Amazon
- HC-05 Bluetooth Module ……………………… Amazon
- Arduino Board …………………………………….. Amazon
- Breadboard and Jump Wires ………………… Amazon
*Please note: These are affiliate links. I may make a commission if you buy the components through these links.I would appreciate your support in this way!
Basic MAX7219 Arduino Code
Once we connect the modules we are ready to take a look at the Arduino code of the first example. We will use the MaxMatrix library which can be downloaded from GitHub.
- /*
- 8x8 LED Matrix MAX7219 Example 01
- by Dejan Nedelkovski, www.HowToMechatronics.com
- Based on the following library:
- GitHub | riyas-org/max7219 https://github.com/riyas-org/max7219
- */
- #include <MaxMatrix.h>
- int DIN = 7; // DIN pin of MAX7219 module
- int CLK = 6; // CLK pin of MAX7219 module
- int CS = 5; // CS pin of MAX7219 module
- int maxInUse = 1;
- MaxMatrix m(DIN, CS, CLK, maxInUse);
- char A[] = {4, 8,
- B01111110,
- B00010001,
- B00010001,
- B01111110,
- };
- char B[] = {4, 8,
- B01111111,
- B01001001,
- B01001001,
- B00110110,
- };
- char smile01[] = {8, 8,
- B00111100,
- B01000010,
- B10010101,
- B10100001,
- B10100001,
- B10010101,
- B01000010,
- B00111100
- };
- char smile02[] = {8, 8,
- B00111100,
- B01000010,
- B10010101,
- B10010001,
- B10010001,
- B10010101,
- B01000010,
- B00111100
- };
- char smile03[] = {8, 8,
- B00111100,
- B01000010,
- B10100101,
- B10010001,
- B10010001,
- B10100101,
- B01000010,
- B00111100
- };
- void setup() {
- m.init(); // MAX7219 initialization
- m.setIntensity(8); // initial led matrix intensity, 0-15
- }
- void loop() {
- // Seting the LEDs On or Off at x,y or row,column position
- m.setDot(6,2,true);
- delay(1000);
- m.setDot(6,3,true);
- delay(1000);
- m.clear(); // Clears the display
- for (int i=0; i<8; i++){
- m.setDot(i,i,true);
- delay(300);
- }
- m.clear();
- // Displaying the character at x,y (upper left corner of the character)
- m.writeSprite(2, 0, A);
- delay(1000);
- m.writeSprite(2, 0, B);
- delay(1000);
- m.writeSprite(0, 0, smile01);
- delay(1000);
- m.writeSprite(0, 0, smile02);
- delay(1000);
- m.writeSprite(0, 0, smile03);
- delay(1000);
- for (int i=0; i<8; i++){
- m.shiftLeft(false,false);
- delay(300);
- }
- m.clear();
- }
Description: So first we need to include the MaxMatrix.h library, define the pins to which the module is connected, set how many modules we use and define the MaxMatrix object.
For displaying characters we need to define them in an array of characters or bytes, and here I have several examples. We can notice how the bits are forming the characters which are actually zeros and ones. In this case they are rotated 90 degrees but the library example suggests to use them in such a way so that would be easier later to implement the shiftLeft custom function for scrolling a text.
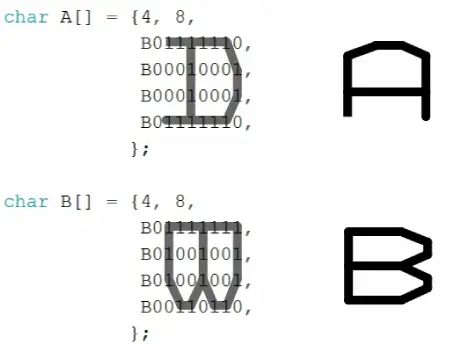
In the setup section we just need to initialize the module and set the brightness of the LEDs. In the loop section using the setDot() function we can set any individual LED to light up at X, Y or Row/ Column position and using the clear() function we can clear the display.
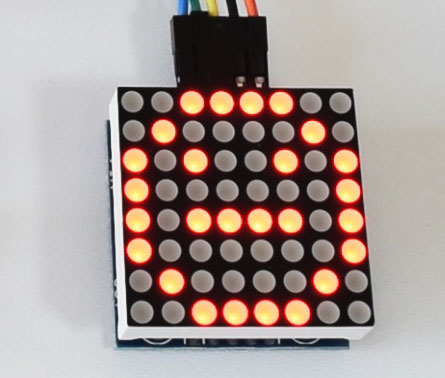
For displaying the predefined characters we use the writeSprite() function, and first two arguments are the X and Y position of the upper left corner of character. At the end using the shiftLeft() function we move or scroll the character to the left.
8×8 LED Matrix Scrolling Arduino Code
Next let’s take a look at the scrolling text example and see what’s different. Below the code you will find its description.
- /*
- 8x8 LED Matrix MAX7219 Scrolling Text Example
- Based on the following library:
- GitHub | riyas-org/max7219 https://github.com/riyas-org/max7219
- */
- #include <MaxMatrix.h>
- #include <avr/pgmspace.h>
- PROGMEM const unsigned char CH[] = {
- 3, 8, B00000000, B00000000, B00000000, B00000000, B00000000, // space
- 1, 8, B01011111, B00000000, B00000000, B00000000, B00000000, // !
- 3, 8, B00000011, B00000000, B00000011, B00000000, B00000000, // "
- 5, 8, B00010100, B00111110, B00010100, B00111110, B00010100, // #
- 4, 8, B00100100, B01101010, B00101011, B00010010, B00000000, // $
- 5, 8, B01100011, B00010011, B00001000, B01100100, B01100011, // %
- 5, 8, B00110110, B01001001, B01010110, B00100000, B01010000, // &
- 1, 8, B00000011, B00000000, B00000000, B00000000, B00000000, // '
- 3, 8, B00011100, B00100010, B01000001, B00000000, B00000000, // (
- 3, 8, B01000001, B00100010, B00011100, B00000000, B00000000, // )
- 5, 8, B00101000, B00011000, B00001110, B00011000, B00101000, // *
- 5, 8, B00001000, B00001000, B00111110, B00001000, B00001000, // +
- 2, 8, B10110000, B01110000, B00000000, B00000000, B00000000, // ,
- 4, 8, B00001000, B00001000, B00001000, B00001000, B00000000, // -
- 2, 8, B01100000, B01100000, B00000000, B00000000, B00000000, // .
- 4, 8, B01100000, B00011000, B00000110, B00000001, B00000000, // /
- 4, 8, B00111110, B01000001, B01000001, B00111110, B00000000, // 0
- 3, 8, B01000010, B01111111, B01000000, B00000000, B00000000, // 1
- 4, 8, B01100010, B01010001, B01001001, B01000110, B00000000, // 2
- 4, 8, B00100010, B01000001, B01001001, B00110110, B00000000, // 3
- 4, 8, B00011000, B00010100, B00010010, B01111111, B00000000, // 4
- 4, 8, B00100111, B01000101, B01000101, B00111001, B00000000, // 5
- 4, 8, B00111110, B01001001, B01001001, B00110000, B00000000, // 6
- 4, 8, B01100001, B00010001, B00001001, B00000111, B00000000, // 7
- 4, 8, B00110110, B01001001, B01001001, B00110110, B00000000, // 8
- 4, 8, B00000110, B01001001, B01001001, B00111110, B00000000, // 9
- 2, 8, B01010000, B00000000, B00000000, B00000000, B00000000, // :
- 2, 8, B10000000, B01010000, B00000000, B00000000, B00000000, // ;
- 3, 8, B00010000, B00101000, B01000100, B00000000, B00000000, // <
- 3, 8, B00010100, B00010100, B00010100, B00000000, B00000000, // =
- 3, 8, B01000100, B00101000, B00010000, B00000000, B00000000, // >
- 4, 8, B00000010, B01011001, B00001001, B00000110, B00000000, // ?
- 5, 8, B00111110, B01001001, B01010101, B01011101, B00001110, // @
- 4, 8, B01111110, B00010001, B00010001, B01111110, B00000000, // A
- 4, 8, B01111111, B01001001, B01001001, B00110110, B00000000, // B
- 4, 8, B00111110, B01000001, B01000001, B00100010, B00000000, // C
- 4, 8, B01111111, B01000001, B01000001, B00111110, B00000000, // D
- 4, 8, B01111111, B01001001, B01001001, B01000001, B00000000, // E
- 4, 8, B01111111, B00001001, B00001001, B00000001, B00000000, // F
- 4, 8, B00111110, B01000001, B01001001, B01111010, B00000000, // G
- 4, 8, B01111111, B00001000, B00001000, B01111111, B00000000, // H
- 3, 8, B01000001, B01111111, B01000001, B00000000, B00000000, // I
- 4, 8, B00110000, B01000000, B01000001, B00111111, B00000000, // J
- 4, 8, B01111111, B00001000, B00010100, B01100011, B00000000, // K
- 4, 8, B01111111, B01000000, B01000000, B01000000, B00000000, // L
- 5, 8, B01111111, B00000010, B00001100, B00000010, B01111111, // M
- 5, 8, B01111111, B00000100, B00001000, B00010000, B01111111, // N
- 4, 8, B00111110, B01000001, B01000001, B00111110, B00000000, // O
- 4, 8, B01111111, B00001001, B00001001, B00000110, B00000000, // P
- 4, 8, B00111110, B01000001, B01000001, B10111110, B00000000, // Q
- 4, 8, B01111111, B00001001, B00001001, B01110110, B00000000, // R
- 4, 8, B01000110, B01001001, B01001001, B00110010, B00000000, // S
- 5, 8, B00000001, B00000001, B01111111, B00000001, B00000001, // T
- 4, 8, B00111111, B01000000, B01000000, B00111111, B00000000, // U
- 5, 8, B00001111, B00110000, B01000000, B00110000, B00001111, // V
- 5, 8, B00111111, B01000000, B00111000, B01000000, B00111111, // W
- 5, 8, B01100011, B00010100, B00001000, B00010100, B01100011, // X
- 5, 8, B00000111, B00001000, B01110000, B00001000, B00000111, // Y
- 4, 8, B01100001, B01010001, B01001001, B01000111, B00000000, // Z
- 2, 8, B01111111, B01000001, B00000000, B00000000, B00000000, // [
- 4, 8, B00000001, B00000110, B00011000, B01100000, B00000000, // \ backslash
- 2, 8, B01000001, B01111111, B00000000, B00000000, B00000000, // ]
- 3, 8, B00000010, B00000001, B00000010, B00000000, B00000000, // hat
- 4, 8, B01000000, B01000000, B01000000, B01000000, B00000000, // _
- 2, 8, B00000001, B00000010, B00000000, B00000000, B00000000, // `
- 4, 8, B00100000, B01010100, B01010100, B01111000, B00000000, // a
- 4, 8, B01111111, B01000100, B01000100, B00111000, B00000000, // b
- 4, 8, B00111000, B01000100, B01000100, B00101000, B00000000, // c
- 4, 8, B00111000, B01000100, B01000100, B01111111, B00000000, // d
- 4, 8, B00111000, B01010100, B01010100, B00011000, B00000000, // e
- 3, 8, B00000100, B01111110, B00000101, B00000000, B00000000, // f
- 4, 8, B10011000, B10100100, B10100100, B01111000, B00000000, // g
- 4, 8, B01111111, B00000100, B00000100, B01111000, B00000000, // h
- 3, 8, B01000100, B01111101, B01000000, B00000000, B00000000, // i
- 4, 8, B01000000, B10000000, B10000100, B01111101, B00000000, // j
- 4, 8, B01111111, B00010000, B00101000, B01000100, B00000000, // k
- 3, 8, B01000001, B01111111, B01000000, B00000000, B00000000, // l
- 5, 8, B01111100, B00000100, B01111100, B00000100, B01111000, // m
- 4, 8, B01111100, B00000100, B00000100, B01111000, B00000000, // n
- 4, 8, B00111000, B01000100, B01000100, B00111000, B00000000, // o
- 4, 8, B11111100, B00100100, B00100100, B00011000, B00000000, // p
- 4, 8, B00011000, B00100100, B00100100, B11111100, B00000000, // q
- 4, 8, B01111100, B00001000, B00000100, B00000100, B00000000, // r
- 4, 8, B01001000, B01010100, B01010100, B00100100, B00000000, // s
- 3, 8, B00000100, B00111111, B01000100, B00000000, B00000000, // t
- 4, 8, B00111100, B01000000, B01000000, B01111100, B00000000, // u
- 5, 8, B00011100, B00100000, B01000000, B00100000, B00011100, // v
- 5, 8, B00111100, B01000000, B00111100, B01000000, B00111100, // w
- 5, 8, B01000100, B00101000, B00010000, B00101000, B01000100, // x
- 4, 8, B10011100, B10100000, B10100000, B01111100, B00000000, // y
- 3, 8, B01100100, B01010100, B01001100, B00000000, B00000000, // z
- 3, 8, B00001000, B00110110, B01000001, B00000000, B00000000, // {
- 1, 8, B01111111, B00000000, B00000000, B00000000, B00000000, // |
- 3, 8, B01000001, B00110110, B00001000, B00000000, B00000000, // }
- 4, 8, B00001000, B00000100, B00001000, B00000100, B00000000, // ~
- };
- int DIN = 7; // DIN pin of MAX7219 module
- int CLK = 6; // CLK pin of MAX7219 module
- int CS = 5; // CS pin of MAX7219 module
- int maxInUse = 2;
- MaxMatrix m(DIN, CS, CLK, maxInUse);
- byte buffer[10];
- char text[]= "HowToMechatronics.com "; // Scrolling text
- void setup() {
- m.init(); // module initialize
- m.setIntensity(15); // dot matix intensity 0-15
- }
- void loop() {
- printStringWithShift(text, 100); // (text, scrolling speed)
- }
- // Display=the extracted characters with scrolling
- void printCharWithShift(char c, int shift_speed) {
- if (c < 32) return;
- c -= 32;
- memcpy_P(buffer, CH + 7 * c, 7);
- m.writeSprite(32, 0, buffer);
- m.setColumn(32 + buffer[0], 0);
- for (int i = 0; i < buffer[0] + 1; i++)
- {
- delay(shift_speed);
- m.shiftLeft(false, false);
- }
- }
- // Extract the characters from the text string
- void printStringWithShift(char* s, int shift_speed) {
- while (*s != 0) {
- printCharWithShift(*s, shift_speed);
- s++;
- }
- }
Labels:
Android,
arduino Projects,
LED Matrix
Thanks for reading LED 8×8 Matrix MAX7219-19 with Scrolling Text AND Android Control via Bluetooth. Please share...!
0 Comment for "LED 8×8 Matrix MAX7219-19 with Scrolling Text AND Android Control via Bluetooth"